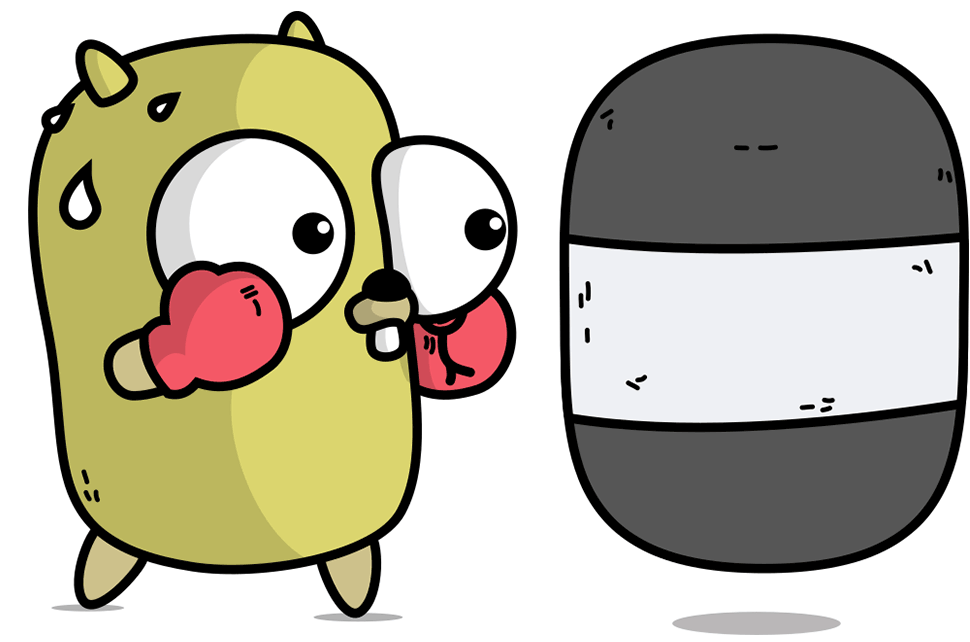
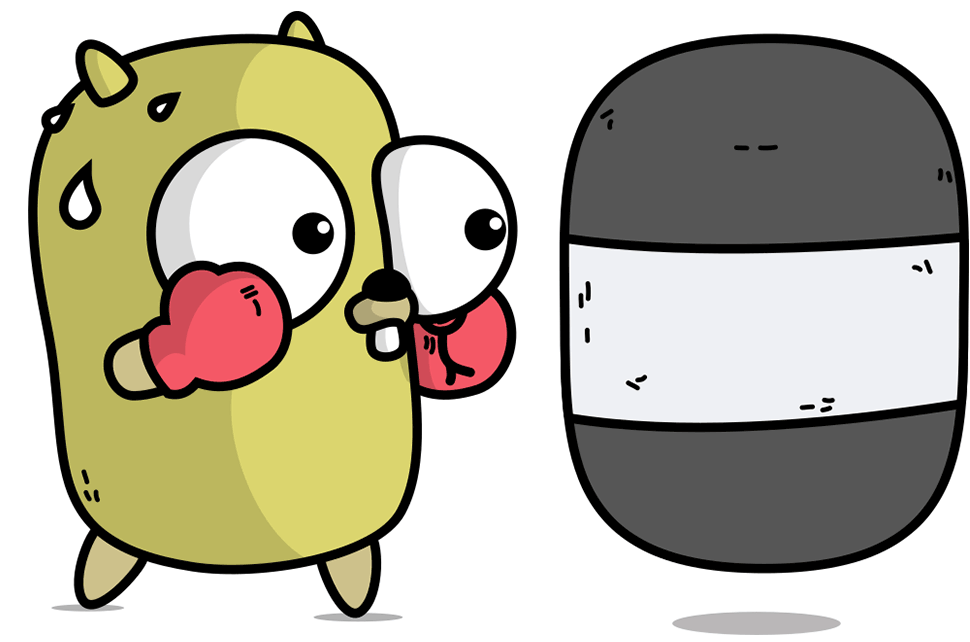
What will we be building?
Note: Sign up to get immediate access to the course and all of the published exercises.
Gophercises is free, but you need to provide a working email address to gain access. I won't spam you and unsubscribing is very easy.
We've all been there before...
You are just starting to pick up a new programming language (like Go!) and things are going great. The tutorials are clicking and you are making great progress... and then you run out of tutorials.
"What should I build next?" you ask, and inevitably everyone tells you to "Pick a side project and work on it!"
Great, but what side project should you work on?
What project will provide you with the best learning experience?
What happens if you pick something too challenging and get stuck?
Nothing sucks more than getting stuck because you didn't know you were tackling a nearly impossible problem, and when you are just getting started how are you supposed to know what those are?
Now take a deep breath and imagine having the confidence to evaluate a project and determine if it was within your grasp.
Imagine knowing that even if you have never used a library before, you will be able to figure it out by reading through the docs.
You can get there, but to do it you need to practice. You need to find projects that will challenge you, but won't leave you clueless about how to proceed.
Enter Gophercises!
Gophercises is a FREE course that will help you become more familiar with Go while developing your skills as a programmer. In the course we will build roughly 20 different mini-applications, packages, and tools that are each designed to teach you something different.
In the course we will learn about and practice using:
By completing the exercises in Gophercises you will slowly become more confident using the Go programming language. You will start to learn how to read the standard docs and make sense of them. You will even start to learn how to evaluate the difficulty of a project before doing much coding. In short, you will start to become a great Go developer.
Note: Sign up to get immediate access to the course and all of the published exercises.
If not, what can I do to convince you? Let me know!